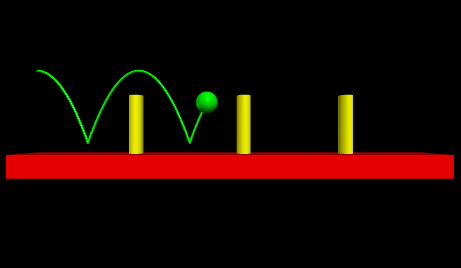
Activity Information
Learning Goals
- Create and modify computational models to describe a given system
- Apply projectile motion concepts
Prior Knowledge Required
- Scalar and Vector quantities
- Kinematics in 2 dimensions
Code Manipulation
- Interpreting existing code
- Modifying existing code
- Synthesize new code from existing code
- Copy/paste code
Activity
Handout – Bounce
Your team has invented some new rubber ball substance that has a coefficient of restitution equal to 1.00!
Your task is to model the bouncing behavior of one of these special new toys as it bounces over some obstacles. Your ball should have some non-zero initial horizontal velocity. The model must include a surface from which to bounce and at least three equal size obstacles over which it must bounce (see sample illustration). Have your bouncing ball leave a trail so the path may be examined.
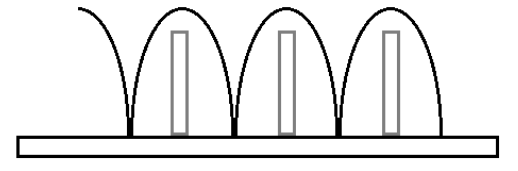
Code
GlowScript 2.7 VPython
#the box defined below is the surface from which the ball will bounce.
#you will need to expand it horizontally and add some obstacles for the ball to bounce over.
surface = box(pos=vector(0,-10,0), size=vector(15,8,15), color=color.red)
ball = sphere(pos=vector(0,24,0), radius=4, color=color.green)
#g, of course, is the acceleration due to gravity.
g = vector(0,-9.8,0)
#below is the initial velocity of the ball.
#currently it is zero--that is, the ball is simply being dropped.
#you must give it an appropriate initial horizontal velocity.
ballv = vector(0,0,0)
t = 0
dt = 0.02
#in the while loop, the ball position is updated by updating the velocity.
#the velocity is updated due to the acceleration due to gravity.
#the ball falls until the bottom of the ball strikes the top of the surface.
# that is the "if" statement
while ball.pos.y < 25:
rate(120)
if ball.pos.y>-2:
ballv = ballv+g*dt
ball.pos = ball.pos + ballv*dt
#once the ball strikes the surface, the "down" velocity becomes "up" velocity
# that is the "else" statement
#then we go back to the top of the while loop and let g continue to update the velocity
else:
ballv.y = -ballv.y
ball.pos = ball.pos + ballv*dt
Answer Key
Handout
The size of the surface the ball bounces on needs to be increased, an initial velocity for the ball in the x-direction needs to be given, and the obstacles for the ball to bounce over need to added.
Optionally, a way to stop the simulation after the last bounce can be added as well.
To make the surface the ball bounces on larger its size object property can be changed:
surface = box(pos=vector(0,-10,0), size=vector(150,8,15), color=color.red)
To give the ball some initial velocity in the x-direction, line 18 can be changed:
ballv = vector(8,0,0)
The x coordinates of the 3 obstacles can be chosen simply by trying different numbers until 3 that work are found, or they can be calculated:
First, we need to find how long it takes the ball to fall from its max height. This will come from the kinematic equation: d = (vi)t + 1/2at^2. Noting that our initial velocity in the y-direction is 0 m/s, this equation can be re-written:
d = 1/2at^2 => h = 1/2gt^2 => t = sqrt(2y/g)
This is the same time that it will take the ball to get back to its max height after bouncing! Now we need to find how far the ball goes in the x-direction in twice this time (because the ball starts at its max height). This can be found with the constant velocity equation:
v = d/t => d = vt
Adding this distance to the initial x position of the ball will give the x coordinate where the ball it at its highest point after its first bounce, which is the optimal location for one of the obstacles.
Adding example obstacles to the code:
color=color.green)
obs1 = cylinder(pos=vector(-34,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
obs2 = cylinder(pos=vector(5,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
obs3 = cylinder(pos=vector(42,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
Finally, the code should stop running at some point. There are a few different ways to add this to the model. For example, the condition statement could be changed so it runs until the ball reaches a certain x coordinate. This solution counts how many times the ball has bounced and stops the code after four bounces.
Code
GlowScript 2.7 VPython
#the box defined below is the surface from which the ball will bounce.
#you will need to expand it horizontally and add some obstacles for the ball to bounce over.
surface = box(pos=vector(0,-10,0), size=vector(150,8,15), color=color.red)
ball = sphere(make_trail=True, pos=vector(-70,24,0), radius=4, color=color.green)
obs1 = cylinder(pos=vector(-34,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
obs2 = cylinder(pos=vector(5,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
obs3 = cylinder(pos=vector(42,-10,0), axis=vector(0,25,0), radius = 2.5, color = color.yellow)
bounces = 0
#g, of course, is the acceleration due to gravity.
g = vector(0,-9.8,0)
#below is the initial velocity of the ball.
#currently it is zero--that is, the ball is simply being dropped.
#you must give it an appropriate initial horizontal velocity.
ballv = vector(8,0,0)
t = 0
dt = 0.02
#in the while loop, the ball position is updated by updating the velocity.
#the velocity is updated due to the acceleration due to gravity.
#the ball falls until the bottom of the ball strikes the top of the surface.
# that is the "if" statement
while ball.pos.y < 25:
rate(120)
if ball.pos.y>-2:
ballv = ballv+g*dt
ball.pos = ball.pos + ballv*dt
#once the ball strikes the surface, the "down" velocity becomes "up" velocity
# that is the "else" statement
#then we go back to the top of the while loop and let g continue to update the velocity
else:
ballv.y = -ballv.y
ball.pos = ball.pos + ballv*dt
bounces = bounces + 1 # this counts up a new variable each time the ball bounces
if bounces == 4:
break # once the ball lands a 4th time, stop the program