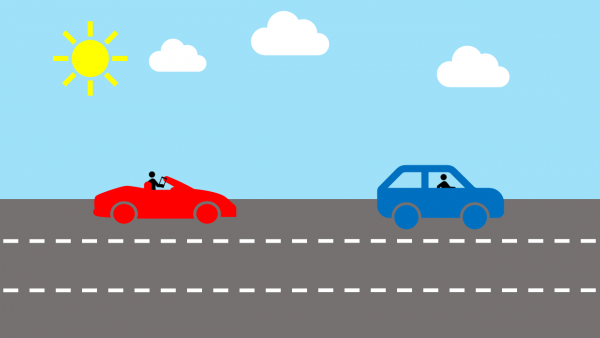
Activity Information
Learning Goals
- Apply the principles of constant velocity motion ( HS-PS2-1)
- Create and modify computational models to describe/show a given system
Prior Knowledge Required
- 1-Dimensional kinematics
- The relationship between position, distance, displacement, speed, and velocity
- Position vs. time and velocity vs. time graphs
- Scalar and vector quantities
Code Manipulation
- Copying/pasting code
- Creating/using while loops
- Converting mathematical equations into code
Activity
Handout – Rear-end Collision
You are driving on the freeway and there is a blue car next to you in the left lane. The blue car is driving at a constant velocity. You notice a red car in your rear-view mirror in the left lane a certain distance behind the blue car. The red car appears to be going very fast and the driver is also looking down, likely texting. If the red car driver continues to text and remains oblivious to the blue car in their lane, how much time does the driver of the blue car have to notice and take evasive cation before they are rear-ended?
- When and where will the crash occur?
- Create a position vs. time graph that includes both cars’ motion (optional).
Note: when conducting this activity with students, the initial positions and velocities of the vehicles can be provided by the instructor, but for demonstration purposes they have been included in the code below.
To perform this virtual experiment in real life, constant velocity toy cars can be purchased here.
Code
GlowScript 2.7 VPython
## Code for a particle object and a red buggy and a blue buggy *Do not change!*
#assume buggy positions are in meters
redbuggy = box(pos=vector(-5,1,0), length = 2, width = 1, height = 1,color = color.red)
bluebuggy = box(pos=vector(0,1,0), length = 2, width = 1, height = 1,color = color.blue)
ground = box(pos=vector(0,0,0), length = 20, width = 1, height = 1, color=color.white)
##Get your red/fast buggy and determine its velocity and define it in the redbuggy x vector below
##take your red/fast buggy back to your teacher
##Get your blue/slow buggy and determine its velocity and define it in the bluebuggy x vector below
##take your blue/slow buggy back to your teacher
redbuggy.velocity = vector(5,0,0)
bluebuggy.velocity = vector(3,0,0)
## Set up the time variables for the while loop for a tf that seems to make sense
dt = 0.01
t = 0
tf = 5
#a function to show accumulated time *Do not change!*
def add_time(t,dt):
new_t = t+dt
return new_t
#Code for a way to start the program after a person clicks on screen *Do not change!*
scene.waitfor('click')
##Code for a "while loop" to make the program iterate over the time interval *Do not change!*
while redbuggy.pos.x < bluebuggy.pos.x-2:
## Define the rate at which the program runs the loop *Do not change!*
rate(100)
## Write a way for the program to update position of each buggy based on its velocity *Do not change!*
redbuggy.pos = redbuggy.pos + redbuggy.velocity*dt
bluebuggy.pos = bluebuggy.pos + bluebuggy.velocity*dt
#define how the program will calculate time for each iteration *Do not change!*
t=add_time(t,dt)
#create a way for the program to show the time and postitions of the buggies *Do not change!*
print("time=",t,"RedPos = ",redbuggy.pos.x,"BluePos = ", bluebuggy.pos.x)
#Use the program to determine how you will know when and where your buggies will collide!
Answer Key
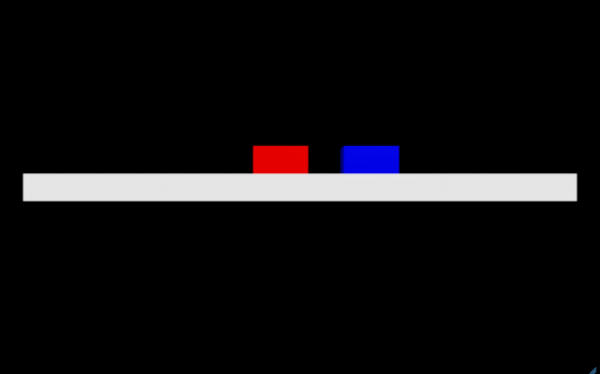
Handout
We can solve for where and when the crash will occur by creating a system of equations and solving (much like we did in the Head-On Collision activity). Let’s start by creating a kinematic equation describing the motion of the fast, red buggy: We know the initial position of the red buggy is 5 units to the left of the origin (line 4) and moving to the right with a velocity of 5 units or length per unit of time (line 12). Therefore, the kinematic equation to describe the position of the red buggy is x=−5+5t. Now let’s do the same for the slower, blue buggy: x=0+3t (lines 5 and 13). Setting these equations equal to each other will allow us to find out how long until the collisions: −5+5t=0+3t. However, we aren’t quite ready to solve first; We need to remember that these buggies have a physical length of 2 (lines 3 and 4) while their positions in the code represent the centers of the buggies. We don’t want to solve for when the cars are intersecting but rather when the front of the red buggy collides with the rear bumper of the blue buggy. We can compensate for this in the equations by adding a length unit for the red buggy equation and subtracting a length unit for the blue buggy equation: x=−4+5t and x=−1+3t, respectively. Now we can solve for t by setting the equations equal to one-another:
-4 + 5t = 1 + 3t
Simplifying we get 2t=3 therefore t=3/2=1.5. The crash will occur after 1.5 units of time. We can plug this back into any of our two initial kinematic equations to find out where the crash will occur:
x = −4 + (5 ∗ 1.5) = −1 + (3 ∗ 1.5) = 3.5
.The buggies will collide 3.5 units to the right of the origin; in other words (accounting for the length of each vehicle), the position of the center of the red buggy at the crash site will be at 2.5 units to the right of the origin and the the center of the blue buggy will be at 4.5 units.
See bolded lines below to see important code modifications.
Code
GlowScript 2.7 VPython
## Code for a particle object and a ground object
redbuggy = box(pos=vector(-5,1,0), length = 2, width = 1, height = 1,color = color.red)
bluebuggy = box(pos=vector(0,1,0), length = 2, width = 1, height = 1,color = color.blue)
ground = box(pos=vector(0,0,0), length = 20, width = 1, height = 1, color=color.white)
## Assign the particle a fixed velocity
redbuggy.velocity = vector(5,0,0)
bluebuggy.velocity = vector(3,0,0)
## Creates an arrow to visualize the particle's velocity
vArrow = arrow(pos=redbuggy.pos, axis = redbuggy.velocity, color = redbuggy.color)
vArrow = arrow(pos=bluebuggy.pos, axis = bluebuggy.velocity, color = bluebuggy.color)
## Set up the time variables for the while loop
dt = 0.01
t = 0
tf = 5
def add_time(t,dt):
new_t = t+dt
return new_t
#Click Screen to Start
scene.waitfor('click')
## While loop to iterate over the time interval
while redbuggy.pos.x < bluebuggy.pos.x-2:
rate(100) ## Defines the rate at which the program runs
## Update position of particle based on its velocity
redbuggy.pos = redbuggy.pos + redbuggy.velocity*dt
bluebuggy.pos = bluebuggy.pos + bluebuggy.velocity*dt
#redbuggy.pos
## Keep the arrow representing the particle's velocity and acceleration
vArrow.pos = redbuggy.pos
vArrow.axis = redbuggy.velocity
vArrow.pos = bluebuggy.pos
vArrow.axis = bluebuggy.velocity
t=add_time(t,dt)
print("time=",t,"RedPos = ",redbuggy.pos.x,"BluePos = ", bluebuggy.pos.x)