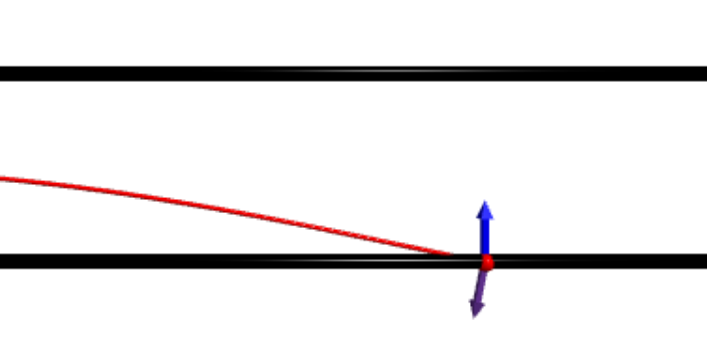
Activity Information
Learning Goals
- Develop a deeper understanding of the Magnetic Force and the Electrostatic Force
- Develop a computational model of a Wien filter
- Learn about current experimental physics
Prior Knowledge Required
- Vectors
- Magnetic Force
- Electrostatic Force
Code Manipulation
- Interpret existing code
- Create code from Mathematical equations
- Copy/paste code
Activity
Handout – Wien Filter
You and your team have been hired as a particle physicist at the definitely not made-up Location for Investigative Accelerator Research (LIAR). Some scientists at LIAR have been working on constructing a device called a Wien filter but they aren’t sure how the physics behind it works. This is where your team comes in. You’ve been tasked with finishing this simulation of a Wien filter. Before working on the code, however, you’ll need to know some background information on what Wien filters are:
Background Information
In the real world, Wien filters, or velocity filters, are commonly used in particle separators, such as SECAR at FRIB, the St. George Separator at the University of Notre Dame, and CRIB at the Center for Nuclear Study. Their purpose is to filter out particles moving at undesired velocities by deflecting them.
Within a Wien filter, there are constant electric and magnetic fields that are orthogonal (perpendicular) to each other and the path of particles passing through the filter. Running the starter code should help visualize this, the arrows represent the B and E fields.
Pre-coding question:
There is a certain velocity called the Wien filter condition at which the beam particles will experience no net force, and thus won’t be deflected. Working in variables (E,B,v,q etc.), what is the value of the Wien filter condition?
While you’re working on the code, information found here might be useful.
Post-coding questions:
- What Force is being ignored in this problem, why is it okay to do so?
- Are the values being used in this problem accurate to what are used in actual nuclear physics research?
- Set the velocity of the particle to the Wien filter condition. Do you observe what you expect?
Code
GlowScript 3.1 VPython
# set the view - you might need to adjust these for your screen
scene.center = vec(0,0,0)
scene.range = 4.5
scene.background = color.white
# Define constant B and E fields
B = vec(0,0,1)
E = vec(0,1,0)
# Object Setup
guide1 = cylinder(pos = vector(-10,1.5,0),axis = vector(20,0,0), radius=0.12, color = color.black) # Visual aid to make it easy to see deflection
guide2 = cylinder(pos = vector(-10,-1.5,0),axis = vector(20,0,0), radius=0.12, color = color.black)
particle = sphere(pos = vec(-8,0,0), radius = 0.15, m = 0.5, v = vec(0,0,0), q = 0.1, color = color.red, make_trail = True) # what we are sending through the filter
# Arrows
Barrow = arrow(pos = vec(-5,0,0), axis = B, color = color.orange) # Arrow to visualize the magnetic field
Earrow = arrow(pos = vec(-5,0,0), axis = E, color = color.cyan) # Arrow to visualize the electric field
FEarrow = arrow(pos = particle.pos, axis = vec(0,0,0), color = color.blue) # Arrow to visualize the magnetic force, will be updated in while loop
FBarrow = arrow(pos = particle.pos, axis = vec(0,0,0), color = color.purple) # Arrow to visualize the electric force, will be updated in while loop
t = 0
dt = 0.1
scale = 10 # scale up the force arrows so they are easy to see
particle.v = vec(1.2,0,0)
while particle.pos.x < 7 and abs(particle.pos.y) < 1.5:
rate(100)
# Calculate forces
FB = vec(0,0,0) # mag force
FE = vec(0,0,0) # electric force
Fnet = vec(0,0,0)
particle.v = particle.v + Fnet/particle.m*dt # Update Particle's Velocity
particle.pos = particle.pos + particle.v*dt # Update Particle's Position
# Update force arrows so we can see the forces on the particle
t = t + dt
Answer Key
Handout
Let’s tackle the pre-coding question first. First, we need to identify what forces are acting on the particle. In this case, the particle has a charge, so it will experience a force from the electric field equal to:
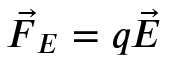
Note that this force will point upward, in the positive y direction.
The particle is also moving in a magnetic field, so it will also experience the magnetic force which is given by:
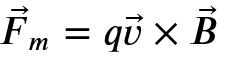
However, because v and B are orthogonal, this expression becomes simply F = qvB. In this case, the right-hand rule tells us that this force will point in the negative y direction, so we will actually say that F = -qvB.
Now we can add these forces together to get the net force:

We want the net force to be zero, which means that E-vB must be zero, which implies that the Wien filter condition is:
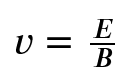
Now we can turn our attention to the code.
First, let’s add the equation for the magnetic force in line 33:
FB = particle.q*cross(particle.v,B)
Then the Electrostatic force in line 34:
FE = E*particle.q
Now we can add the net force in the next line:
Fnet = FB + FE
That’s all that needs to be done to get the physics of the problem to work properly. Now we can add some code to update the force arrows:
# Update arrows
FEarrow.pos = particle.pos
FEarrow.axis = FE*scale
FBarrow.pos = particle.pos
FBarrow.axis = FB*scale
Post-Coding Questions:
- We’re ignoring the force of gravity in this problem. We can do this because the masses of particles in particle accelerators are extremely small, so they don’t experience much force from gravity.
- These values aren’t accurate at all, for example 0.5 kg for the mass of the particle is huge.
- As expected, the particle continues in a straight line, and isn’t deflected at all.
Code
GlowScript 3.1 VPython
# set the view - you might need to adjust these for your screen
scene.center = vec(0,0,0)
scene.range = 4.5
scene.background = color.white
# Define constant B and E fields
B = vec(0,0,1)
E = vec(0,1,0)
# Object Setup
guide1 = cylinder(pos = vector(-10,1.5,0),axis = vector(20,0,0), radius=0.12, color = color.black) # Visual aid to make it easy to see deflection
guide2 = cylinder(pos = vector(-10,-1.5,0),axis = vector(20,0,0), radius=0.12, color = color.black)
particle = sphere(pos = vec(-8,0,0), radius = 0.15, m = 0.5, v = vec(0,0,0), q = 0.1, color = color.red, make_trail = True) # what we are sending through the filter
# Arrows
Barrow = arrow(pos = vec(-5,0,0), axis = B, color = color.orange) # Arrow to visualize the magnetic field
Earrow = arrow(pos = vec(-5,0,0), axis = E, color = color.cyan) # Arrow to visualize the electric field
FEarrow = arrow(pos = particle.pos, axis = vec(0,0,0), color = color.blue) # Arrow to visualize the magnetic force, will be updated in while loop
FBarrow = arrow(pos = particle.pos, axis = vec(0,0,0), color = color.purple) # Arrow to visualize the electric force, will be updated in while loop
t = 0
dt = 0.1
scale = 10 # scale up the force arrows so they are easy to see
particle.v = vec(mag(E)/mag(B)+0.2,0,0)
while particle.pos.x < 7 and abs(particle.pos.y) < 1.5:
rate(100)
# Calculate forces
FB = particle.q*cross(particle.v,B)
FE = E*particle.q
Fnet = FB + FE
particle.v = particle.v + Fnet/particle.m*dt # Update Particle's Velocity
particle.pos = particle.pos + particle.v*dt # Update Particle's Position
# Update arrows
FEarrow.pos = particle.pos
FEarrow.axis = FE*scale
FBarrow.pos = particle.pos
FBarrow.axis = FB*scale
t = t + dt