Activity Information
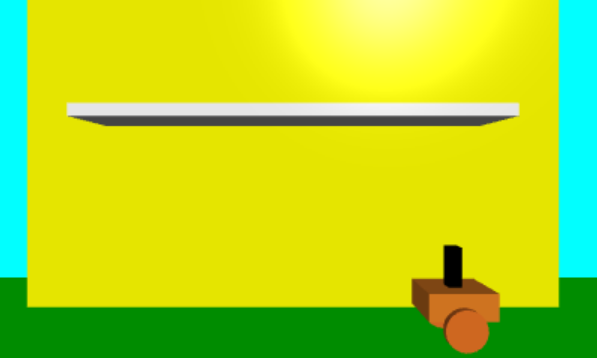
Learning Goals
- Apply the principles of constant acceleration motion in 2d
- Modify a computational model to represent a real-world scenario
Prior Knowledge Required
- Vectors
- Kinematics
Code Manipulation
- Interpret existing code
- Edit existing code
- copy / paste code
Activity
Handout – Zorro’s Escape
In order to escape the villainous Captain Monasterio and his men before they can break into Ana Maria’s house and capture him, Zorro runs upstairs and out onto a balcony. He whistles for his horse and cart and steps off the balcony at the right moment to land in the driver’s seat.
Copy the code below and modify it so that Zorro steps off the balcony at the right moment, falls in a realistic way, lands on the cart and moves with it once he lands on it.
Once you have your code working can you generalize it so it works for other velocities of the cart? For other heights of Zorro?
Code
Link – click “remix” to edit and view the code properly
#Window setup
scene.range = 40
scene.background = color.cyan
#Create the scene
ground = box(pos=vector(0, -30, 0), size=vector(200, 10, 50), color=color.green)
wall = box(pos=vector(0, 10, -10), size=vector(100, 70, 12), color=color.yellow)
balcony = box(pos=vector(0, 10, 2.5), size=vector(70, 2, 13), color=color.white)
zorro = box(pos=vector(0, 15, 8), size=vector(2, 8, 2), color=color.black)
cartbox = box(pos=vector(-40, -19, 10), size=vector(10, 4, 8), color=vector(.9,0.5,.14))
wheel1 = cylinder(pos=vector(-40, -22, 14), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
wheel2 = cylinder(pos=vector(-40, -22, 5), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
cart = compound([cartbox, wheel1, wheel2])
#Setting the initial time and change in time.
t = 0
dt = 0.01
#Giving the objects initial velocity
cartv=vector(10,0,0)
zorrov=vector(0,0,0)
ag = vector(0,-9.8,0)
while cart.pos.x < 60:
rate(200) #DO NOT EDIT THIS LINE. It tells the computer how fast to run the program.
if t < 3:
cart.pos = cart.pos + cartv * dt
else if zorro.pos.y > -15:
cart.pos = cart.pos + cartv * dt
zorro.pos.z = 10 #Zorro steps forward, off the balcony
else:
cart.pos = cart.pos + cartv * dt
t = t + dt
Answer Key
Handout
Finding Times
To find when Zorro needs to jump, we need to first find what time the cart is directly underneath him. we can see from line 10 in the code that the cart starts at x = -40 m and from line 9 we can see that Zorro’s x position is x = 0 m. Line 20 tells us that the velocity of the cart is 10 m/s. To find the time we can use the constant velocity equation:
v = d/t => t = d/v = 4 s
The value of d comes from subtracting the initial position of the cart from the position of the cart when it is directly underneath Zorro.
Now we need to find how long it takes Zorro to fall. To do this we can use the kinematic equation: d = (vi)t + 1/2at^2. Zorro is starting from rest, so vi = 0 m/s. Now the equation can be solved for time:
d = 1/2at^2 => h = 1/2gt^2 => t = sqrt(2y/g)
Our value for y is found by subtracting Zorro’s initial y position (15 m) from his final y position (-15 m), which gives y =-30 m. Plugging in -9.8 m/s^2 for g gives a time to fall of about 2.47 s.
The time that Zorro should jump will be the time it takes the cart to get to x=0 minus the time it takes Zorro to fall, which ends up being about 1.53 s.
Editing Code
Now that we have the time that Zorro should jump, we can add this to the code by changing 3 to 1.53 in line 28. This works because the first if statement represents everything that happens before Zorro jumps.
Now we need to change what’s in the if else statement. We can update Zorro’s position just like the position of the cart is updated:
else if zorro.pos.y > -15:
cart.pos = cart.pos + cartv * dt
zorro.pos = zorro.pos + zorrov * dt
However, this doesn’t do anything because Zorro starts with a velocity of 0, so we will need to update his velocity as well. The velocity update equation is vf = vi + at, which translated into our code will look like:
else if zorro.pos.y > -15:
cart.pos = cart.pos + cartv * dt
zorrov = zorrov + ag * dt
zorro.pos = zorro.pos + zorrov * dt
Finally, we need to make it so that Zorro moves with the cart after he lands in it. This can be done in the else statement. Because Zorro is in the cart, he will have the same velocity as it, so we can update his position using the cart’s velocity:
else:
cart.pos = cart.pos + cartv * dt
zorro.pos = zorro.pos + cartv * dt
Generalizing the code
To generalize the code, we need to have it calculate the time that Zorro should jump like we did by hand before. This means putting those equations into code form:
#calculating the time to jump
cartt = -cart.pos.x / cartv.x
fallt = sqrt(2*(-15 - zorro.pos.y) / ag.y )
jumpt = cartt - fallt
This can be calculated before the while loop. In the while loop, we now need to change the time of the condition statement for the first while loop to jumpt:
while cart.pos.x < 60:
rate(200)
if t < jumpt:
cart.pos = cart.pos + cartv * dt
Code
Specific solution:
Link – click “remix” to edit and view the code properly
#Window setup
scene.range = 40
scene.background = color.cyan
#Create the scene
ground = box(pos=vector(0, -30, 0), size=vector(200, 10, 50), color=color.green)
wall = box(pos=vector(0, 10, -10), size=vector(100, 70, 12), color=color.yellow)
balcony = box(pos=vector(0, 10, 2.5), size=vector(70, 2, 13), color=color.white)
zorro = box(pos=vector(0, 15, 8), size=vector(2, 8, 2), color=color.black)
cartbox = box(pos=vector(-40, -19, 10), size=vector(10, 4, 8), color=vector(.9,0.5,.14))
wheel1 = cylinder(pos=vector(-40, -22, 14), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
wheel2 = cylinder(pos=vector(-40, -22, 5), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
cart = compound([cartbox, wheel1, wheel2])
#Setting the initial time and change in time.
t = 0
dt = 0.01
#Giving the objects initial velocity
cartv=vector(10,0,0)
zorrov=vector(0,0,0)
ag = vector(0,-9.8,0)
while cart.pos.x < 60:
rate(200) #DO NOT EDIT THIS LINE. It tells the computer how fast to run the program.
if t < 1.53:
cart.pos = cart.pos + cartv * dt
else if zorro.pos.y > -15:
cart.pos = cart.pos + cartv * dt
zorrov = zorrov + ag * dt
zorro.pos = zorro.pos + zorrov * dt
zorro.pos.z = 10 #Zorro steps forward, off the balcony
else:
cart.pos = cart.pos + cartv * dt
zorro.pos = zorro.pos + cartv * dt
t = t + dt
General solution:
Link – click “remix” to edit and view the code properly
#Window setup
scene.range = 40
scene.background = color.cyan
#Create the scene
ground = box(pos=vector(0, -30, 0), size=vector(200, 10, 50), color=color.green)
wall = box(pos=vector(0, 10, -10), size=vector(100, 70, 12), color=color.yellow)
balcony = box(pos=vector(0, 10, 2.5), size=vector(70, 2, 13), color=color.white)
zorro = box(pos=vector(0, 15, 8), size=vector(2, 8, 2), color=color.black)
cartbox = box(pos=vector(-40, -19, 10), size=vector(10, 4, 8), color=vector(.9,0.5,.14))
wheel1 = cylinder(pos=vector(-40, -22, 14), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
wheel2 = cylinder(pos=vector(-40, -22, 5), axis=vector(0, 0, 1), radius = 3, color=vector(.9,0.45,.14))
cart = compound([cartbox, wheel1, wheel2])
#Setting the initial time and change in time.
t = 0
dt = 0.01
#Giving the objects initial velocity
cartv=vector(13,0,0)
zorrov=vector(0,0,0)
ag = vector(0,-9.8,0)
#calculating the time to jump
cartt = -cart.pos.x / cartv.x
fallt = sqrt(2*(-15 - zorro.pos.y) / ag.y )
jumpt = cartt - fallt
while cart.pos.x < 60:
rate(200) #DO NOT EDIT THIS LINE. It tells the computer how fast to run the program.
if t < jumpt:
cart.pos = cart.pos + cartv * dt
else if zorro.pos.y > -15:
cart.pos = cart.pos + cartv * dt
zorrov = zorrov + ag * dt
zorro.pos = zorro.pos + zorrov * dt
zorro.pos.z = 10 #Zorro steps forward, off the balcony
else:
cart.pos = cart.pos + cartv * dt
zorro.pos = zorro.pos + cartv * dt
t = t + dt